Example BASIC program
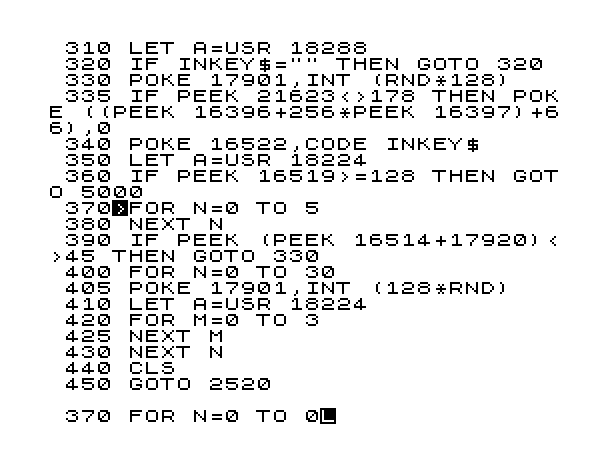
Here’s an example ZX Spectrum Basic program that solves a quadratic equation:
10 PRINT “Quadratic Equation Solver”
20 PRINT “Enter the coefficients:”
30 INPUT “a: “, a
40 INPUT “b: “, b
50 INPUT “c: “, c
60 LET d = b*b – 4ac
70 IF d < 0 THEN 80 PRINT “No real solutions”
90 ELSE IF d = 0 THEN
100 LET x = -b / (2a)
110 PRINT “One real solution: x =”; x
120 ELSE
130 LET x1 = (-b + SQR(d)) / (2a)
140 LET x2 = (-b – SQR(d)) / (2a)
150 PRINT “Two real solutions:”
160 PRINT “x1 =”; x1
170 PRINT “x2 =”; x2
180 END IF
190 PRINT “End of program”
This program prompts the user to enter the coefficients (a, b, and c) of a quadratic equation in the form of ax^2 + bx + c = 0. It then calculates the discriminant (d) to determine the number and nature of the solutions.
If the discriminant is less than 0, the program prints “No real solutions” since the equation has no real roots. If the discriminant is equal to 0, it calculates the single solution and displays it. If the discriminant is greater than 0, it calculates and displays the two real solutions.
Finally, the program prints “End of program” to indicate the completion of the execution.
You can type and run this program on a ZX Spectrum computer or in an emulator to solve quadratic equations.